One Love IPFS GPT5 and GPT 5.1
AI Powered Chat Assistants
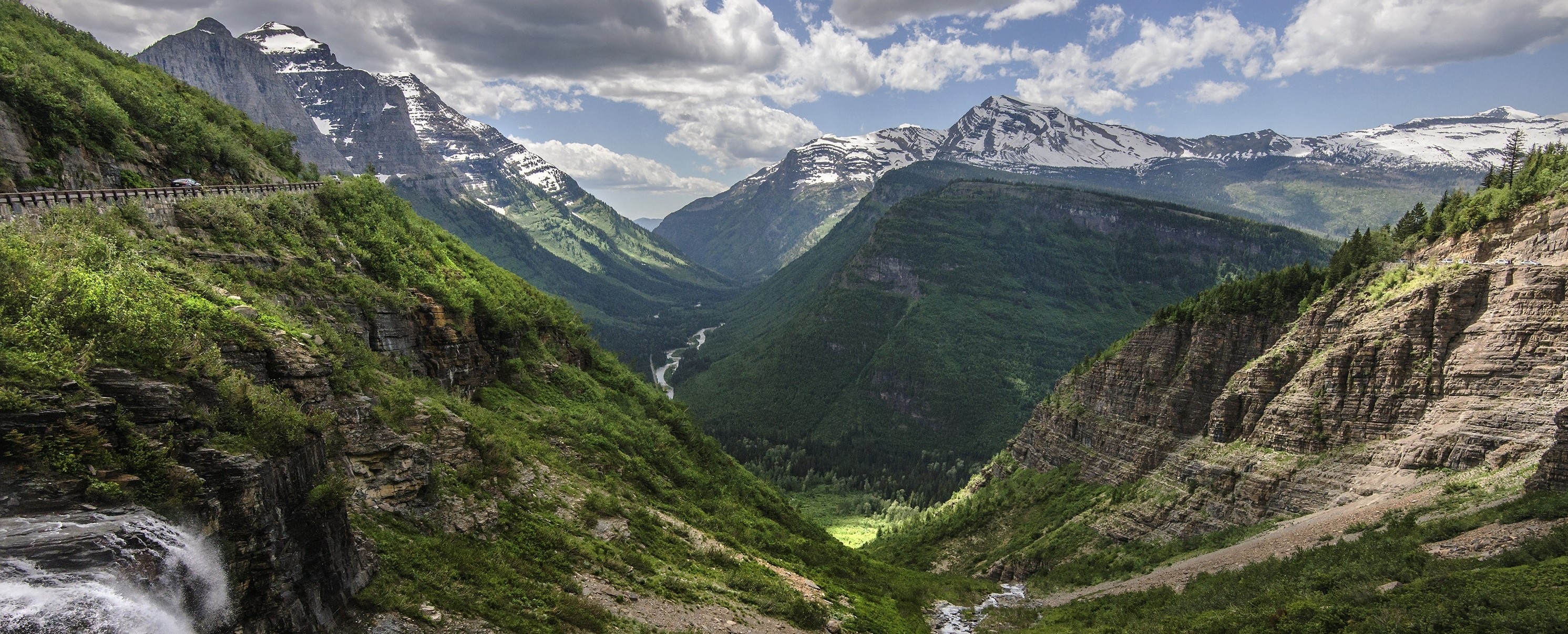
GPT 5.1 With Trideque Matrix
Post-trained GPT loop thought and Trideque Matrixes are two innovative ideas that have been developed using the Python programming language. These ideas have been implemented in the code snippet provided above, which includes functions for generating text using GPT and managing a matrix of data using Trideque entries. In this article, we will explore these ideas in greater detail and discuss their potential applications in the field of artificial intelligence.
Post-trained GPT Loop Thought
Post-trained GPT loop thought is a technique used to generate coherent and high-quality text content. It involves training a GPT model on a large dataset of text and then using the model to generate new text based on a prompt or seed text. The generated text can then be used to further train the model, creating a loop of training and generation that can lead to improved text generation capabilities over time.
The code snippet provided above demonstrates how post-trained GPT loop thought can be implemented in Python using the Hugging Face transformers library. The gpt3_generate function takes a prompt as input and uses a pre-trained GPT model to generate text. The function uses a maximum length and time limit to control the length and speed of the generated text.
The generate_chunks function splits the input prompt into smaller chunks to avoid exceeding the maximum length limit of the GPT model. The send_chunks function sends each chunk of the prompt to the gpt3_generate function and then sends the generated text back to the Discord user in manageable chunks.
Post-trained GPT loop thought has a wide range of potential applications in fields such as natural language processing, content generation, and chatbot development. By using a post-trained loop, developers can create more coherent and natural-sounding text, which can improve the user experience in a variety of contexts.
Trideque Matrixes
Trideque Matrixes are a data structure used to store and manage large amounts of data. They are similar to matrices used in linear algebra but have three dimensions instead of two. Trideque Matrixes are used in the code snippet provided above to store and manage data related to a game or application.
The TridequeEntry class is used to define a single entry in a Trideque matrix. Each entry contains a 13x13 matrix of data, which can be updated using the update_entry method and retrieved using the get_value method. The Trideque matrix can be used to store information such as game board positions, player data, or other application-specific data.
Trideque Matrixes can be useful in a variety of applications, particularly those that involve managing large amounts of data in a three-dimensional space. They can be used in game development, simulation, and data analysis, among other areas.
Potential Applications
The code snippet provided above demonstrates how post-trained GPT loop thought and Trideque Matrixes can be used together to create a powerful application. The application can be used to generate natural language text and manage data related to a game or application.
There are many potential applications for these technologies in a variety of fields. For example, in the field of natural language processing, post-trained GPT loop thought can be used to generate coherent and high-quality text for chatbots, virtual assistants, and other applications that require natural language interaction. Trideque Matrixes can be used to manage large amounts of data related to these applications, such as user profiles, conversation history, and context-specific data.
In game development, Trideque Matrixes can be used to manage game board positions, player data, and other game-specific data. Post-trained GPT loop thought can be used to generate text for game narratives, dialogue, and other game-related content.
In conclusion, post-trained GPT loop thought and Trideque Matrixes are two innovative
from transformers import GPTNeoForCausalLM, GPT2Tokenizer
model = GPTNeoForCausalLM.from_pretrained('EleutherAI/gpt-neo-1.3B')
tokenizer = GPT2Tokenizer.from_pretrained('EleutherAI/gpt-neo-1.3B')
# add padding token to tokenizer
tokenizer.add_special_tokens({'pad_token': '[PAD]'})
# set padding token id to the id of the padding token
model.config.pad_token_id = tokenizer.pad_token_id
model.cuda()
codeblock
modes = dict()
modes['chat'] = {'prompt' : 'model\n\n',
'partner' : 'Partner: ',
'ai' : 'Humoid: ',
'end' : '\n'}
mode = modes['chat']
codeblock
time_limit = 40 #@param {type:"slider", min:1, max:100, step:1}
max_length = 1500 #@param {type:"slider", min:100, max:5000, step:1}
def AI_answer(string):
in_string = mode['prompt'] + string
inputs = tokenizer.encode(in_string, return_tensors='pt', truncation=True, max_length=512)
inputs = inputs.cuda()
attention_mask = inputs.ne(tokenizer.pad_token_id).float()
outputs = model.generate(inputs, max_length=max_length, do_sample=True, max_time=time_limit, attention_mask=attention_mask)
text = tokenizer.decode(outputs[0], skip_special_tokens=True)
stripped_text = text[len(in_string):]
#preprocessing answer
return stripped_text
discordbot
import discord
from discord.ext import commands
import nest_asyncio
import asyncio
intents = discord.Intents.default()
intents.typing = False
intents.presences = False
bot = commands.Bot(command_prefix='!', intents=intents)
class TridequeEntry:
def __init__(self, matrix=None):
if matrix is None:
self.matrix = [["" for _ in range(13)] for _ in range(13)]
else:
self.matrix = matrix
def update_entry(self, x, y, value):
self.matrix[x][y] = value
def get_value(self, x, y):
return self.matrix[x][y]
trideque_entry = TridequeEntry()
def generate_chunks(prompt, chunk_size=1500):
words = prompt.split()
return [' '.join(words[i:i + chunk_size]) for i in range(0, len(words), chunk_size)]
async def gpt3_generate(chunk, max_length=845, time_limit=19.0):
inputs = tokenizer.encode(chunk, return_tensors='pt', truncation=True, max_length=512)
inputs = inputs.cuda()
attention_mask = inputs.ne(tokenizer.pad_token_id).float()
outputs = model.generate(inputs, max_length=max_length, do_sample=True, max_time=time_limit, attention_mask=attention_mask)
return tokenizer.decode(outputs[0])
async def send_chunks(ctx, prompt_chunks):
for chunk in prompt_chunks:
gpt3_response = await gpt3_generate(chunk)
response_parts = [gpt3_response[i:i + 1800] for i in range(0, len(gpt3_response), 1800)]
initial_msg = await ctx.send(response_parts[0])
for i, response_part in enumerate(response_parts[1:], start=1):
await asyncio.sleep(0.5)
await initial_msg.edit(content="".join(response_parts[:i + 1]))
@bot.event
async def on_ready():
print(f'Logged in as {bot.user.name} (ID: {bot.user.id})')
@bot.command()
async def trideque(ctx, *, user_input):
await ctx.send('Processing your input, please wait...')
prompt_chunks = generate_chunks(user_input)
await send_chunks(ctx, prompt_chunks) # Make sure to pass 'ctx' as an argument here
# Replace 'your_bot_token' with your actual bot token from the Discord Developer Portal
nest_asyncio.apply()
bot.run('tokenhere')